Jenkinsfile 语法进阶
在编写 Jenkinsfile 时,不要将其看成有固定套路的编排语言,而 Jenkinsfile 本身就是 Groovy 进行编写,而 Groovy 有一些语法需要学习,但是他和 Java 的语法是通用的。
我们每一个插件都是一个函数,插件的参数就是函数的参数。
我们同样可以在 Jenkinsfile 中进行 for 循环、条件表达式、解析 JSON、对对象进行赋值,遍历数组。
同样的图形编排可能比较简单,但是如果需要实现一个复杂的 CI 流程,还是需要知道如何进行文本编辑的。
模板字符串
- 单引号(’ ‘):在单引号中定义的字符串是字面量,它们被视为静态字符串,不会进行变量替换或转义字符的处理。
- 双引号(” “):在双引号中定义的字符串允许变量替换和转义字符的使用。
- 三个单引号(’’’ ‘’’):在三个单引号之间定义的字符串可以跨越多行,保留原始格式。这种字符串通常用于多行文本或代码块
- 三个双引号(””” “””):与三个单引号类似,三个双引号之间定义的字符串也可以跨越多行,并保留原始格式。它们与三个单引号的区别在于,三个双引号中的字符串可以包含变量替换和转义字符。
pipeline {
agent any
stages {
stage("test") {
steps {
script {
def name = 'John'
println('Hello, ' + name + '!') // 输出:Hello, John!
println("Hello, $name!") // 输出:Hello, John!
println("Hello, ${name}!") // 输出:Hello, John!
}
}
}
stage("test2") {
steps {
script {
def message = '''
This is a multi-line
string in Groovy.
'''
println(message)
// Copy
// This is a multi-line
// string in Groovy.
def name = 'John'
def tmp = """
Hello, $name!
Hello,${name}
"""
println(tmp) // 输出:Hello, John!
}
}
}
}
}
希望通过例子可以了解到,变量 var 被双引号包起来"$var"
"${var}"
,被三个双引号包起来的行为 """${var}"""
"""$var"""
。
所以很多时候使用模板字符串不是必须的,如下面这个例子。
pipeline {
agent any
stages {
stage("test") {
steps {
script {
def name = 'John'
echo name // 输出: John
echo "$name" // 输出: John
echo "${name}" // 输出: John
}
}
}
}
}
插件的参数
你可以将 Jenkins 的插件理解为一个函数,插件的参数就是函数的参数列表。
上面一个小结中我们了解到了 echo
这个函数。
源码地址: https://github.com/jenkinsci/workflow-basic-steps-plugin。
官方文档地址: https://www.jenkins.io/doc/pipeline/steps/workflow-basic-steps/#echo-print-message。
他有且仅有一个参数,参数的名字叫做 message
。
pipeline {
agent any
stages {
stage("test") {
steps {
script {
def name = 'John'
echo name // 输出: John
echo(name) // 输出: John
echo(message: name) // 输出: John
}
}
}
}
}
插件的返回值
pipeline {
agent any
stages {
stage("test") {
steps {
script {
def name = sh script: "echo John",returnStdout: true
echo name // 输出: John
echo(sh(script: "echo John",returnStdout: true))
}
}
}
}
}
环境变量与 map
在一次构建中,用户配置的环境变量和系统默认生成的环境变量都是挂载在 env
这个 map 上的,groovy 的结构是 def env = [:]
。
环境变量的使用方式,以变量名 BRANCH_NAME 举例:
- 直接使用 BRANCH_NAME
- env.BRANCH_NAME
- env[“BRANCH_NAME”]
- env 是全局变量,可以在多个作用域使用,将需要传递的值挂载到
env
上
pipeline {
agent any
stages {
stage("test") {
steps {
echo BRANCH_NAME
echo env.BRANCH_NAME
echo env["BRANCH_NAME"]
script{
env.A = sh(script: "echo HelloWorld",returnStdout: true)
}
}
}
stage("test2") {
steps {
echo env.A
}
}
}
}
自定义函数
def sum(int a, int b){
return a + b;
}
pipeline {
agent any
stages {
stage("test") {
steps {
script {
echo sum(1,2).toString()
}
}
}
}
}
if 条件判断
def sum(int a, int b){
return a + b;
}
pipeline {
agent any
stages {
stage("test") {
steps {
script {
if (sum(1,2).equals(3)){
echo "true"
} else {
echo "false"
}
if (env.BRANCH_NAME == 'master') {
echo 'I only execute on the master branch'
} else {
echo 'I execute elsewhere'
}
}
}
}
}
}
when 表达式
pipeline {
agent any
stages {
stage('Example Build') {
steps {
echo 'Hello World'
}
}
stage('Example Deploy') {
when {
expression { BRANCH_NAME ==~ /(production|staging)/ }
}
steps {
echo 'Deploying'
}
}
}
}
for 和 each 循环
pipeline {
agent any
stages {
stage('Example Build') {
steps {
echo 'Hello World'
}
}
stage('Example Deploy') {
steps {
script {
[1,2,3,4].each {
print "this is each ${it}"
}
for(int i=0;i<10;i++){
print "this is for ${i}"
}
}
}
}
}
}
通过 JSON 环境变量生成 stage step
import groovy.json.JsonSlurperClassic
def json(jsonString){
def dataObject = new JsonSlurperClassic().parseText(jsonString)
return dataObject
}
def parseStages(s){
def jsonData = json(s)
return jsonData.collect{ item ->
return stage(item.name){
item.steps.each{ step->
sh(script: step)
}
}
}
}
node {
stage("123"){
sh "echo 123"
}
parseStages(env.jsonstring)
}
传入一个环境变量 envstring
[ { "name": "stage1", "steps": [ "echo 123", "echo 456" ] }, { "name": "stage2", "steps": [ "echo helloworld2", "echo helloworld###" ] }, { "name": "stage3", "steps": [ "echo 3", "echo 33333" ] } ]
动态生成并行构建任务
def tasks = [
"echo 1",
"echo 2",
"echo 3"
]
def stages = [
failFast: true,
"后端构建": {
tasks.each { task ->
stage("build ${task}") {
sh task
}
}
}
]
node{
parallel(stages)
}
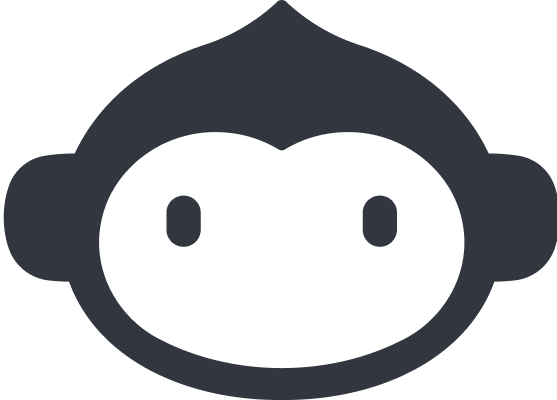
在阅读中是否遇到以下问题?*
您希望我们如何改进?*
如果您希望得到回复,请留下您的邮箱地址。